I knew starting this assignment that I wanted to work with squares, because I like the type of grid pattern they can create.
I considered doing Random Squares William Kolomyjec, as shown below:
but instead I did this piece that has been done by various people. Here is the link: http://recodeproject.com/artwork/v1n4untitled-1
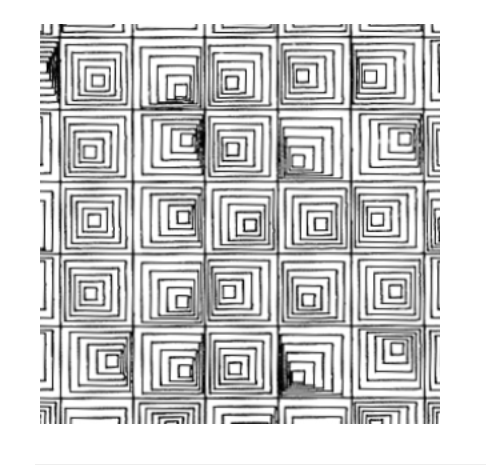
Here is my version:
I think it would have looked better if I had kept my screen smaller, as in the example piece, but I wanted mine to be full screen while still keeping the same number of rows and columns.
Here is my code:
static final int cols = 11; // as the image showed 11 columns
static final int rows = 10; // as the image showed 10 rows
void setup() {
fullScreen();
noLoop();
}
void draw() {
rectMode(CENTER); // draws from the center to make it easier. Thank goodness for this.
strokeWeight(2);
fill(255);
int squareSize= 140;
int sizeDiff = 20;
float offsetX;
float offsetY;
for (int r = 0; r<rows; r++) // runs 10 times for 10 rows
{
for (int c = 0; c<cols; c++) // runs 11 times for 11 columns
{
offsetX = random(-5, 5);// will get a new random x each time
offsetY = random(-5, 5);// will get a new random y each time
rect(c*squareSize, r*squareSize, squareSize, squareSize); //draws the outermost squares, all the same size just in different positions
for (int n=1; n<6; n++) // runs 5 times as each square has 5 squares inside
{
rect((c*squareSize)+(n*offsetX), // takes the column/x value of my square and offsets it
(r*squareSize)+(n*offsetY), // takes the row/y value of my square and offsets it
squareSize – (n*sizeDiff), //makes the square smaller and smaller
squareSize – (n*sizeDiff));
}
}
}
}