For my selfportrait, I wanted to go a bit more abstract in my design. It is truly, Picasso inspired.
This was a lot of fun to make, as I went really colorful and used a lot of abstract shapes.
Here is a photo that inspired me.
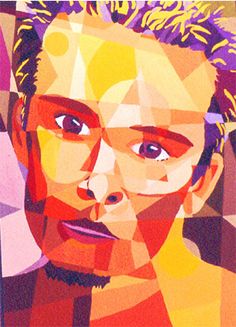
And so, my picture looks like this:
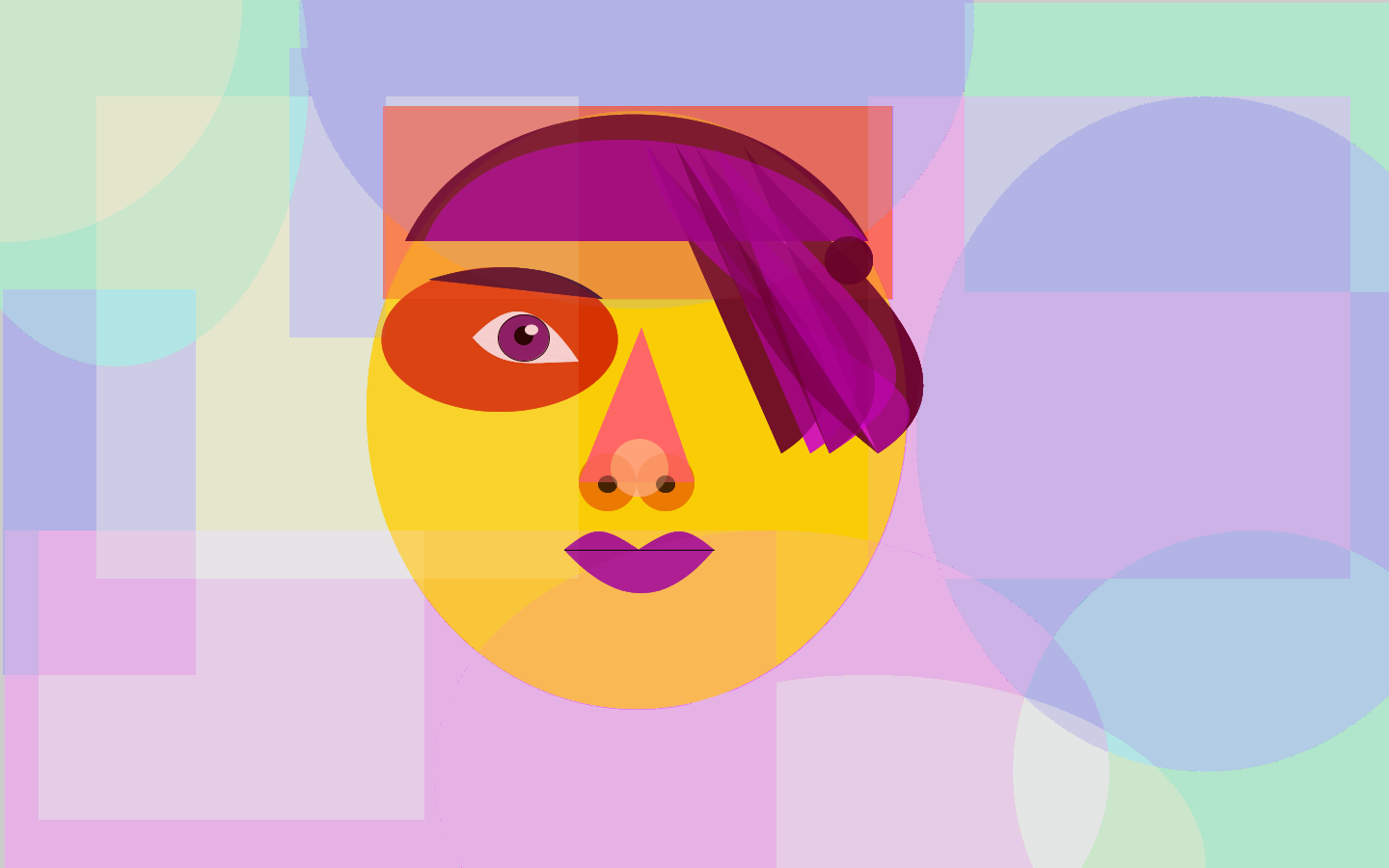
Here is my code:
void setup(){
fullScreen();
}
void draw(){
//peach color of skin with full opacity
//fill(240,220,200,255);
//yellow color face
fill(255, 204, 0, 20);
//draw the face outline
ellipse(660,425, 560, 620);
//forhead color
fill(255, 92, 51, 20);
//draw forehead
rect(397, 110, 528, 200);
//get rid of the color
noFill();
//top outline eye
bezier(488, 348, 518, 318, 548, 298, 600, 373);
//bottom outline eye
bezier(490, 352, 522, 387, 552, 377, 602, 377);
//get rid of the lines
//——————————–EVERYTHING PAST THIS WILL HAVE NO OUTLINE——————————————————
noStroke();
//idk what color this is
// fill(0,220,200,20);
//white color for eyes with full opacity
fill(255,255,255,255);
//inner eyeball that is now white; top part
bezier(490, 350, 520, 320, 550, 300, 600, 375);
//inner eyeball that is now white; bottom part
bezier(490, 350, 520, 385, 550, 375, 600, 375);
//color for the iris as dark purple
fill(102,0,102,220);
//draw the iris
ellipse(543, 350, 52, 48);
//(for the eyeball/pupil) black with full transparency
fill(0,0,0,255);
//draw the pupil
ellipse(543, 348, 20, 20);
//——————————–OUTLINE——————————————————
//sets the stroke to black /line for the iris details and iris outline
stroke(0);
noFill();
//outline for the iris
ellipse(543, 350, 53.5, 48);
noStroke();
//——————————–EVERYTHING PAST THIS WILL HAVE NO OUTLINE——————————————————
//sets the color to white for glare
fill(255,255,255,255);
//draw glare
ellipse(551, 342, 14, 11);
//sets color for eyebrows
fill(77, 25, 51);
//draws the left eyebrow
bezier(445,290, 490, 275, 570, 265, 625, 310);
//sets the red ellipse shadow for eye under brow
fill(204, 0 ,0, 50);
//draws the ellipse under the eye
ellipse(518, 352, 245, 150);
//sets the color for the nose
fill(255, 102, 102, 255);
//draws the triangle for the nose
//rect(635, 395, 55, 80, 3, 6, 12, 18);
triangle(600, 500, 720, 500, 665, 340);
//nose tip color
fill(255, 179, 128, 200);
//draw the nose tip
ellipse(663,485, 60,60);
//left nostril color
fill(230, 92, 0, 50);
//draw left nostril
ellipse(630, 500, 60,60);
//draw right nostril
ellipse(690, 500, 60, 60);
//nose hole
fill(0,0,0, 100);
//draw the holes
ellipse(630, 502, 20,18);
ellipse(690, 502, 20,18);
//lip color
fill(153, 0, 153, 100);
//draw the top lip
bezier(662, 570, 700, 545, 710, 545, 740, 570);
bezier(585, 570, 615, 545, 625, 545, 662, 570);
//draw the bottom of the lip
bezier(585, 570, 640, 630, 690,630, 740, 570);
//——————————–OUTLINE——————————————————
stroke(0);
//part of the mouth
line(585, 570, 740, 570);
noFill();
noStroke();
//——————————–EVERYTHING PAST THIS WILL HAVE NO OUTLINE——————————————————
//color for the hair
fill(102, 0, 41, 120);
//begin the hair
bezier(670, 150, 740, 250, 950, 380, 810, 470);
bezier(770, 150, 840, 250, 1050, 380, 910, 470);
//contrast color hair
fill(204, 0, 204, 90);
//hair multistrand1
bezier(670, 150, 700, 350, 1050, 380, 910, 470);
//hair multistrand2
bezier(700, 150, 770, 250, 980, 380, 840, 470);
//color for the hair (again)
fill(102, 0, 41, 120);
bezier(720, 150, 790, 250, 1000, 380, 860, 470);
//contrast color hair
fill(204, 0, 204, 90);
bezier(740, 150, 910, 350, 1000, 380, 860, 470);
//color for the hair
fill(102, 0, 41, 120);
//begin the hair
bezier(420, 250, 500, 80, 800, 70, 900, 250);
//contrast color hair
fill(204, 0, 204, 90);
bezier(440, 250, 500, 100, 800, 120, 900, 250);
//color for the hair
fill(102, 0, 41, 120);
//hair multistrand3
bezier(700, 150, 780, 350, 800, 380, 910, 470);
ellipse(880, 270, 50,50);
ellipse(880, 270, 50,50);
//background fill
fill(255, 255, 204, 5);
ellipse(1,1,500,500);
rect(100, 100, 500,500);
ellipse(900,900, 700, 400);
rect(40, 550, 400,300);
fill(255, 153, 255, 5);
ellipse(800,800,700,500);
rect(900, 100, 500,500);
rect(5, 550, 800,800);
fill(153, 153, 255,5);
ellipse(660,20, 700, 600);
rect(300,50, 100, 300);
rect(3, 300, 200, 400);
ellipse(1250, 450, 600, 700);
fill(153, 255, 204, 5);
rect(1000,3,700, 300);
ellipse(120, 80, 400, 600);
ellipse(1300, 800, 500, 500);
fill(204, 255, 153, 5);
rect(50, 1000, 500, 500);
}