In the world of graphic design . . .
Designers have to constantly work with color values. While it is easy to simply google the R,G,B value of a color, it is a nice skill to recognize what values will output what colors. I thought this would be a fun concept to explore visually, and so the RGB GAME controller emerged.
For this project, there is an input of 3 numbers, ranging from 0 – 255 as the Red, Green and Blue values. (Meaning 0,0,0 will be black and 255,255,255 will be white.) I thought it would be a fun way for people to practice visually looking at colors and relating them to a numerical value (such as that used in coding.)
From there, the numbers are inputed through 3 knobs on the box/controller shown below.
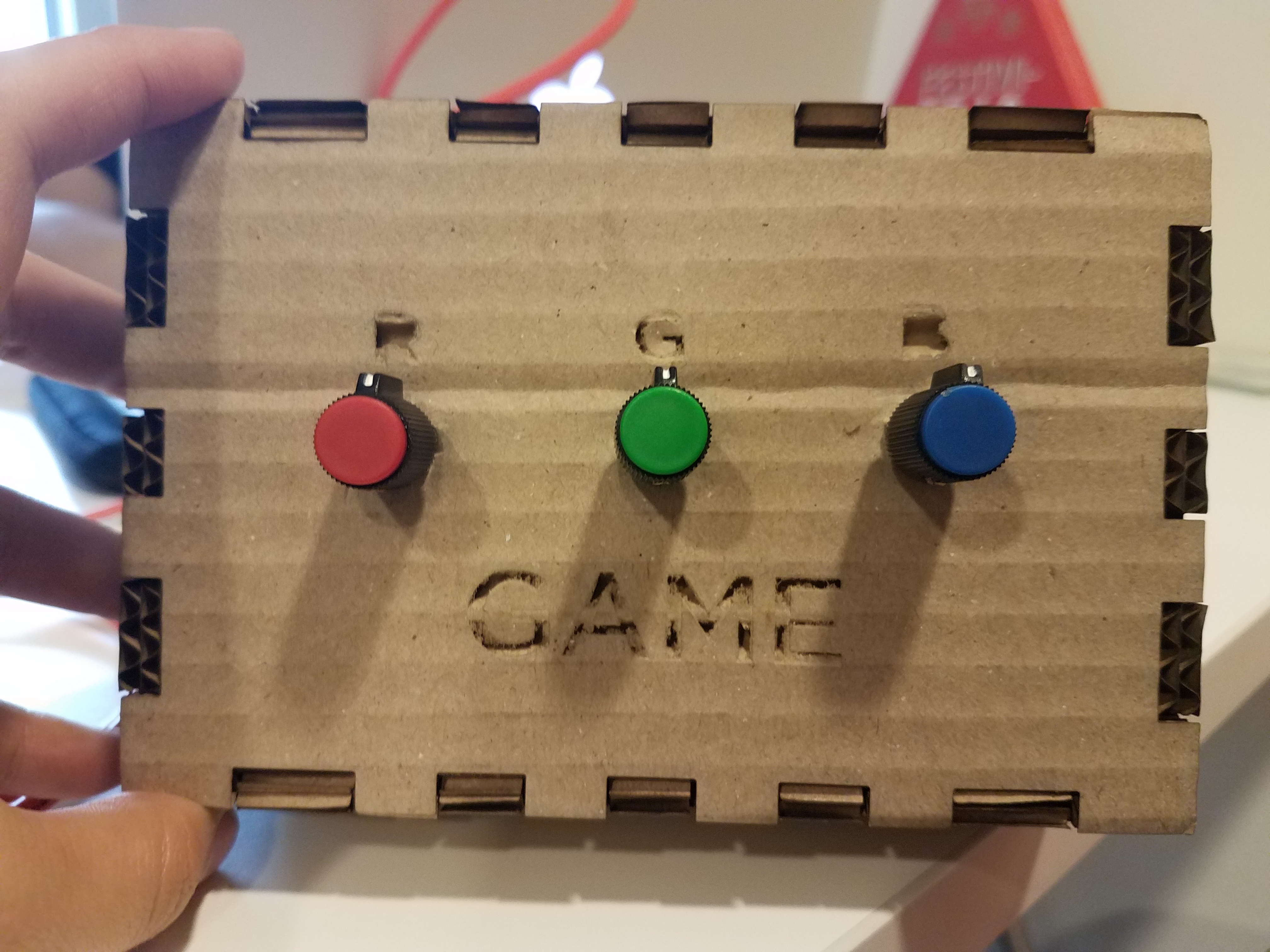
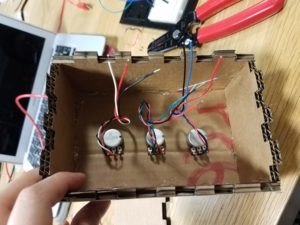
When the game actually begins, the player must turn the knobs in order to try their best to match the color of a randomly drawn rectangle on the screen. The values of the r,g,b values the user is altering are shown on the screen, along with a timer that counts down from 25 seconds.
Originally, I wanted the randomized rectangle that was shown on the screen to disappear after 5 seconds, and the user still had to match the new rectangle from memory. This feature was discarded after some user testing that determined this would make the game a bit too difficult.
Here is a final product video.
How the code works:
There is a range of numbers ( + or – 25) in which the code is checking to see if the random rectangle matches. There is also the timer which will display a loss if the colors are not matched after 25 seconds; a win will be shown immediately.
Here is the code:
import processing.serial.*; PFont font; Serial myPort; String[] messages; int r; int g; int b; boolean complete = false; int begin; int duration = 25; int time = 25; int baseRectR = floor(random(0, 255)); int baseRectG = floor(random(0, 255)); int baseRectB = floor(random(0, 255)); void setup () { //println(Serial.list()); font = createFont("Arial", 40); textFont(font); begin = millis(); printArray(Serial.list()); String portname=Serial.list()[3]; println(portname); myPort = new Serial(this, portname, 9600); fullScreen(); } void draw() { background(255); noStroke(); String vals =r + " " + g + " " + b; String valsSet = "This rect was: " + baseRectR + " " + baseRectG + " " + baseRectB; String valYours = "Your rect was: " + r + " " + g + " " + b; //checks if the colors match within a range of 25 if ( (r-baseRectR <= 25) && (r-baseRectR >= -25) && (g-baseRectG <= 25) && (g-baseRectG >= -25) && (b-baseRectB <= 25) && (b-baseRectB >= -25)) { complete = true; font = createFont("Arial", 75); textFont(font); fill(0); text("YOU HAVE WON :)", 400, height/2); font = createFont("Arial", 40); textFont(font); text(valsSet, 800, 800); text(valYours, 250, 800); noLoop(); } // if colors do not match after the timer is up == lose if (time <=0 && complete == false) { vals = null; fill(0); text("SORRY HOMIE, BETTER LUCK NEXT TIME :(", 250, height/2); font = createFont("Arial", 40); textFont(font); complete = true; text(valsSet, 800, 800); text(valYours, 250, 800); noLoop(); } //displays end message fill(0); if (complete==false) { text(vals, 250, 800); } if (complete == false) { fill(baseRectR, baseRectG, baseRectB); rect((1.8*width)/3, height/3, 400, 400); } fill(r, g, b); // sets timer if ((time > 0) && complete == false) { rect(width/9, height/3, 400, 400); fill(0); time = duration - (millis() - begin)/1000; text(time, width/2.07, height/2); } } //reads the input void serialEvent(Serial porty) { String message = porty.readStringUntil('\n'); if (message != null) { message = trim(message); messages = message.split(","); //println(message); r = int(messages[0]); g = int(messages[1]); b = int(messages[2]); //println(messages[0], messages[1], messages[2]); } }
Overall, I’m super happy with how this turned out! Even the box for the controller ended up looking really cool.